Best Selling Products
Pattern Introduction Part 2: Improving Design Thinking and Application Development
Nội dung
- 1. Overview of patterns in Pattern design
- 1.1. Pattern Definition:
- 1.2. Why are Patterns important in software development?
- 1.3. Basic components of a Pattern:
- 1.4. Pattern Classification:
- 2. Advanced Creational Patterns:
- 2.1. Abstract Factory:
- 2.2. Builder:
- 2.3. Prototype:
- 2.4. Command:
- 3. Combining Patterns and Best Practices
- 4. Conclusion
Explore the important principles of pattern design, common types of patterns, and how to apply them in modern graphic design.
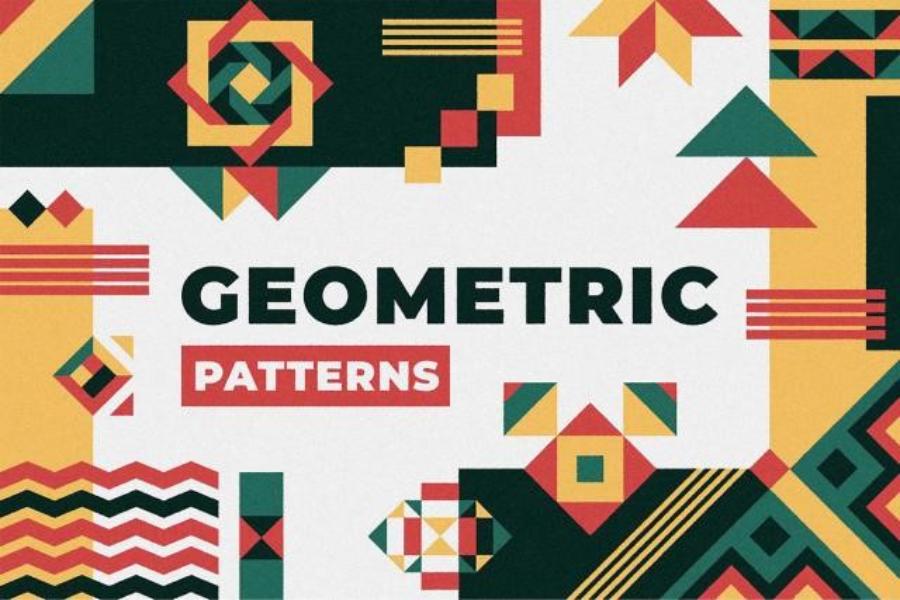
In the fast-paced world of software development, building applications that not only work but are also maintainable, scalable, and flexible is a challenge. Patterns have emerged as proven solutions, providing a framework for solving common design problems. In part 2 of the introductory pattern series, sadesign will continue to explore the core principles, expressive styles, and practical applications of patterns in modern design.
1. Overview of patterns in Pattern design
To make sure we all have a solid foundation before moving on to advanced Patterns , let's review the core knowledge of Patterns .
Genuine Adobe Illustrator Super Cheap Price
1.1. Pattern Definition:
A Pattern or design pattern in software engineering is a general, reusable solution to a common problem in a specific context in software design. It is not a finished design but a blueprint that you can customize to solve your own problem.
1.2. Why are Patterns important in software development?
Reusability: Patterns provide proven solutions, saving you time and effort when solving similar problems.
Common vocabulary: Patterns create a common language among developers, making it easier to communicate and understand design.
Proven solutions: Patterns are often the result of years of experience and have been proven to work in practice.
Improved maintainability and extensibility: Using appropriate Patterns makes the software structure clear, flexible and easy to change as requirements evolve.
Improve software quality: Patterns help avoid common design mistakes and lead to better solutions in terms of performance, reliability, and usability.
1.3. Basic components of a Pattern:
A Pattern is often described as consisting of the following main components:
Pattern Name: A short and memorable name that makes it easy to reference and discuss the Pattern.
Problem: Describes the context and specific design problem that this Pattern solves.
Solution: Describes the components, their relationships, and how they work together to solve the problem. Solutions are often presented as class diagrams or object diagrams.
Consequences: List the benefits and drawbacks of applying the Pattern, as well as its impacts on other aspects of the design (e.g. flexibility, performance, maintainability).
1.4. Pattern Classification:
Patterns are generally classified into three main groups based on their intended use:
Creational Patterns: Concerned with flexible and controlled ways of creating objects. They help hide complex initialization logic and create objects in different ways depending on the situation.
Structural Patterns: Concerned with how classes and objects are combined to create larger and more complex structures. They help define relationships between entities and simplify system structure.
Behavioral Patterns: Concerned with how objects interact and assign responsibilities among them. They help define complex algorithms and control flow.
2. Advanced Creational Patterns:
2.1. Abstract Factory:
Definition and usage: Abstract Factory is a Creational Pattern that provides an interface for creating families of related or interdependent objects without specifying their concrete classes. It allows you to create families of products without knowing in advance the concrete classes that will be used.
Problem solved: When you need to create many different families of objects, and the objects in each family are closely related to each other. Creating these objects directly can lead to complex code that is difficult to change if you want to switch between product families. For example, you may have many different user interfaces (e.g. Windows, macOS), and each of these interfaces consists of many components (e.g. buttons, scrollbars, windows). These components need to be compatible with each other within the same interface.
Structure and participating components:
AbstractFactory: Defines an interface for operations that create all abstract products.
ConcreteFactory: Implements the AbstractFactory interface to create concrete products. Each ConcreteFactory creates a family of concrete products.
AbstractProduct: Defines an interface for a product type.
ConcreteProduct: Implements the AbstractProduct interface. Each ConcreteProduct belongs to a specific product family and is created by a corresponding ConcreteFactory.
Client: Only interacts with the AbstractFactory and AbstractProduct interfaces. It does not know about the concrete Concrete classes.
Advantage:
Isolation of Concrete Layers: Client does not depend on specific product layers.
Easily change product families: Just change the ConcreteFactory used.
Ensure consistency: Products within a family will be compatible with each other.
Disadvantages:
Difficult to add new products: Adding a new product type often requires modifying the AbstractFactory interface and all ConcreteFactories.
May increase the complexity of the original design.
When to use Abstract Factory?
Your system needs to be independent of how its products are created, configured, and performed.
A system consists of several product families, and products in each family need to be used together.
You want to provide a library of product objects that only expose their interface, not their implementation.
2.2. Builder:
Definition and usage: Builder is a Creational Pattern that allows you to build a complex object step by step. This pattern separates the construction of an object from its representation, so the same construction process can produce different representations.
Problem solved: When initializing an object requires many complex steps or has many optional configurations. Having too many constructors with different parameters (telescoping constructors) will make the code difficult to read and maintain. Furthermore, if you want to create different variants of the object with different properties, using traditional constructors will become very complicated.
Structure and participating components:
Builder (Interface): Defines an interface for all the steps to build the parts of the object.
ConcreteBuilder (Concrete class): Implements the Builder interface and provides concrete implementations for each build step. It also manages the creation and return of the final product.
Director (Optional): Contains complex build logic and works with a Builder object to build the product. Clients can interact with the Builder directly or through the Director.
Product: The complex object being built.
Advantage:
Separation of construction and representation: The same construction process can produce different representations.
Construction process control: Construction steps are carried out in a sequential and controlled manner.
Avoid telescoping constructors: Eliminates the need to have multiple constructors with different parameters.
Easily add new build steps.
Disadvantages:
It is possible to increase the number of layers in the system.
Design requirements prior to construction steps.
When to use Builder?
The algorithm for creating a complex object should be independent of the parts that make up that object and how they are assembled.
The construction process allows for the creation of different representations of the object.
You want granular control over the object construction process.
2.3. Prototype:
Definition and usage: Prototype is a Creational Pattern that allows you to create new objects by cloning an existing object, called the prototype. This pattern is useful when creating an instance of a class is expensive or complicated, or when you want to create multiple objects with similar state.
Problem solved: In some cases, creating a new object by calling a constructor can be resource-intensive (e.g., database connection, reading complex configuration). Or, you may want to create multiple objects with the same initial state. The Prototype Pattern solves this problem by allowing you to clone a fully instantiated object.
Structure and participating components:
Prototype (Interface or Abstract Class): Declare an interface for copying methods (usually clone() ).
ConcretePrototype: Implements the Prototype interface and implements the copy method. The copy process can be shallow copy or deep copy depending on the requirement.
Client: Creates new objects by asking the prototype to copy itself.
Advantage:
Reduced initialization costs: Copying an existing object is often faster and less expensive than creating a new one.
Create objects with similar state: Easily create multiple objects with the same initial configuration.
Hides the complexity of object creation: The client does not need to know the details of the initialization process.
Disadvantages:
Implementing deep copies can be complex, especially when the object contains other complex objects with cyclic references.
It can be difficult to copy classes with complex inheritance structures.
When to use Chain of Responsibility?
Multiple objects can handle a request, and the handler is not known in advance.
You want to send a request to one of many objects without explicitly specifying the recipient.
The sequence of request handling objects can be determined dynamically.
2.4. Command:
Definition and usage: Command is a Behavioral Pattern that encapsulates a request as an object, thus allowing you to parameterize clients with queues, requests, and operations. It supports undoable, redoable, and logging operations.
Problem solved: You want to separate the object sending the request (invoker) from the object performing the request (receiver). This allows you to configure the invoker with different commands without knowing the details of the receiver or the operation being performed. It also allows you to perform actions like queuing requests, logging them, and supporting undo/redo.
Structure and participating components:
Command (Interface): Declare an interface for all commands, usually with only one execute() method .
ConcreteCommand (Concrete class): Implements the Command interface by associating a receiver with a specific action. The execute() method calls the receiver's methods.
Invoker (Invoker Object): Stores and requests the execution of Command objects. The Invoker does not know the details about the command or receiver.
Receiver: Performs the action associated with the request. ConcreteCommand delegates the execution to one or more receivers.
Client: Creates ConcreteCommand objects and sets their receivers.
Advantage:
Separate invoker and receiver: Reduce dependencies between objects.
Undo/redo support: Easy to implement by storing history of executed commands.
Logging support: Commands can be logged before and after execution.
Support queuing requests: Commands can be placed in a queue and executed later.
Easily add new commands.
Disadvantages:
It is possible to increase the number of layers in the system.
When to use Command?
You want to parameterize objects with actions.
You want to queue or log requests.
You want to support undoable operations.
You want to separate the object that calls an operation from the object that knows how to perform it.
3. Combining Patterns and Best Practices
Learning and understanding Patterns is just one part of the big picture in software design and development. To truly harness their power, you need to know how to skillfully combine multiple Patterns to solve complex problems. A real-world software system rarely uses just one Pattern. Instead, software architects often combine multiple Patterns to create robust and flexible solutions.
For example, you can use Abstract Factory to create families of objects, and then use Builder to construct complex objects within each family. Or, you can use Observer to notify about state changes, and Command to encapsulate actions that react to those changes.
The relationship between Patterns and object-oriented design principles (SOLID):
Patterns are often concrete implementations of object-oriented design principles, especially the SOLID principles :
Single Responsibility Principle (SRP): Many Patterns help separate responsibilities between classes, such as Command , Strategy , Visitor .
Open/Closed Principle (OCP): Patterns like Strategy , Template Method , Observer , Decorator allow you to extend the behavior of a system without modifying existing code.
Liskov Substitution Principle (LSP): Proper use of interfaces and inheritance in Patterns ensures that subclasses can replace their parent classes without breaking the program.
Interface Segregation Principle (ISP): The pattern focuses on defining small, specific interfaces for each role, avoiding a class having to implement methods it doesn't use.
Dependency Inversion Principle (DIP): Many Creational and Structural Patterns (e.g. Abstract Factory , Dependency Injection - a concept closely related to the Pattern) help reduce dependencies on concrete classes, and instead rely on abstractions.
Notes when applying Pattern (avoid overuse):
It is important to remember that Patterns are not a silver bullet . Blindly applying Patterns without understanding the problem can lead to designs that are too complex and harder to maintain. Only apply Patterns when they truly solve a specific design problem and provide a clear benefit.
The Importance of Understanding the Problem Before Choosing a Pattern:
Before deciding to use a Pattern, make sure you understand the problem you are trying to solve. Analyze the requirements, identify the constraints, and consider other alternative solutions. Only when you are sure that a particular Pattern is the best fit, go ahead and implement it.
4. Conclusion
Mastering these concepts will equip you with a powerful toolkit to tackle complex design challenges in software development. You will be able to build more flexible, maintainable, and scalable applications. Keep learning, practicing, and applying them to your real-world projects. Don’t be afraid to experiment and explore creative combinations of Patterns to create superior software solutions.